Basic logical blocks
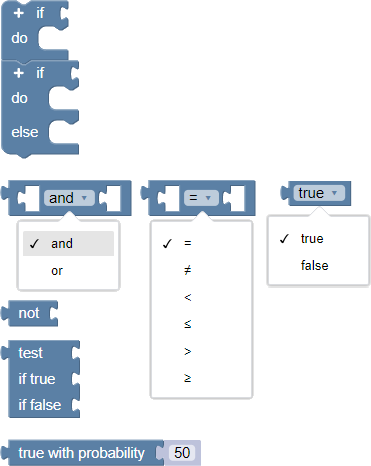
Branch blocks
If condition* is true, perform the action

If condition* is true, perform an action, else perform another action

* Boolean condition - an expression, the result of which is “true” or “false”
You can modify these blocks by adding more conditions to them
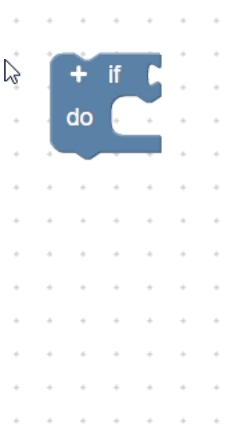
Logic AND and OR
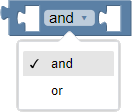
If at least one of the simple statements connected by the AND operation is false, then the compound statement will also be false
If at least one of the simple statements connected by the OR operation is true, then the compound statement will also be true
Comparison operations
Usually used with numbers. Equality operations can also be used on text
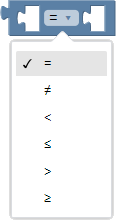
You can only compare data of the same type (you can’t compare numbers and text)
True/False
Boolean variable
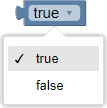
Logical NOT
The logical operation does NOT invert the statement behind it

For example, the following expression (however useless but cognitive) will return true

Ternary conditional operation
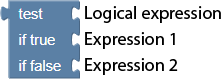
A ternary operation can be defined as an operation that returns its second expression depending on the value of the logical expression given by the first expression. The operation algorithm is as follows:
- The boolean expression is evaluated
- If the logical expression is true, then the value of expression 1 is evaluated; otherwise, the value of expression 2 is evaluated
- The computed value is returned
Only one of the expressions is evaluated: expression 1 or expression 2. This corresponds to the principle of lazy evaluation
Example

- If both simple buttons are pressed, then change “color” to “blue”, otherwise - “red”
- Set a simple light bulb to the color stored in the “color” variable
Which is equivalent to the following construction

True with probability
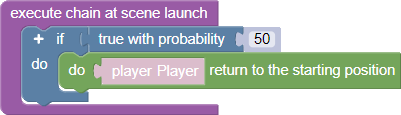
Boolean variable whose truth will depend on the specified probability